Programming with Java: Beginner to Advanced, 7ed (An Indian Adaptation)
ISBN: 9789363865549
For more information write to us at: acadmktg@wiley.com
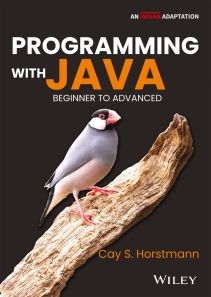
Description
Programming With Java Beginner to Advanced, 7th Edition is a comprehensive guide designed to introduce Java programming to beginners. This edition is tailored for learners with no prior programming experience and requires only a basic understanding of high school algebra. The book employs objects and classes from Java's standard library from the outset, with a structured introduction to object-oriented design commencing in Chapter 8. This gradual approach allows students to familiarize themselves with object usage early on while learning their core algorithmic concepts, thereby avoiding the development of improper coding practices. The latter half of the book covers algorithms and data structures at a level suitable for novice programmers.
PREFACE TO THE ADAPTED EDITION PREFACE SPECIAL FEATURES 1 INTRODUCTION 1.1 Computer Programs 1.2 The Anatomy of a Computer 1.3 The Java Programming Language 1.4 Becoming Familiar with Your Programming Environment 1.5 Analyzing Your First Program 1.6 Errors 1.7 PROBLEM SOLVING: Algorithm Design The Algorithm Concept An Algorithm for Solving an Investment Problem Pseudocode From Algorithms to Programs HT 1 Describing an Algorithm with Pseudocode WE 1 Writing an Algorithm to Estimate the Cost of Painting a House 2 USING OBJECTS 2.1 Objects and Classes Using Objects Classes 2.2 Variables Variable Declarations Types Names Comments Assignment ST 1 Variable Type Inference 2.3 Calling Methods The Public Interface of a Class Method Arguments Return Values Method Declarations 2.4 Constructing Objects 2.5 Accessor and Mutator Methods 2.6 The API Documentation Browsing the API Documentation Packages 2.7 Implementing a Test Program ST 2 Testing Classes in an Interactive Environment WE 1 How Many Days Have You Been Alive? WE 2 Working with Pictures 2.8 Object References 2.9 Graphical Applications Frame Windows Drawing on a Component Displaying a Component in a Frame 2.10 Ellipses, Lines, Text, and Color Ellipses and Circles Lines Drawing Text Colors 3 IMPLEMENTING CLASSES 3.1 Instance Variables and Encapsulation Instance Variables The Methods of the Counter Class Encapsulation 3.2 Specifying the Public Interface of a Class Specifying Methods Specifying Constructors Using the Public Interface Commenting the Public Interface 3.3 Providing the Class Implementation Providing Instance Variables Providing Constructors Providing Methods HT 1 Implementing a Class WE 1 Making a Simple Menu 3.4 Unit Testing 3.5 PROBLEM SOLVING: Tracing Objects 3.6 Local Variables 3.7 The this Reference ST 1 Calling One Constructor from Another 3.8 Shape Classes HT 2 Drawing Graphical Shapes 4 FUNDAMENTAL DATA TYPES 4.1 Numbers Number Types Constants ST 1 Big Numbers 4.2 Arithmetic Arithmetic Operators Increment and Decrement Integer Division and Remainder Powers and Roots Converting Floating-Point Numbers to Integers ST 2 Avoiding Negative Remainders ST 3 Combining Assignment and Arithmetic ST 4 Instance Methods and Static Methods 4.3 Input and Output Reading Input Formatted Output HT 1 Carrying Out Computations WE 1 Computing the Volume and Surface Area of a Pyramid 4.4 PROBLEM SOLVING: First Do it By Hand WE 2 Computing Travel Time 4.5 Strings The String Type Concatenation String Input Escape Sequences Strings and Characters Substrings ST 5 Using Dialog Boxes for Input and Output 5 DECISIONS 5.1 The if Statement ST 1 The Conditional Operator 5.2 Comparing Values Relational Operators Comparing Floating-Point Numbers Comparing Strings Comparing Objects Testing for null HT 1 Implementing an if Statement WE 1 Extracting the Middle 5.3 Multiple Alternatives ST 2 The switch Statement 5.4 Nested Branches ST 3 Block Scope ST 4 Enumeration Types 5.5 PROBLEM SOLVING: Flowcharts 5.6 PROBLEM SOLVING: Selecting Test Cases ST 5 Logging 5.7 Boolean Variables and Operators ST 6 Short-Circuit Evaluation of Boolean Operators ST 7 De Morgan’s Law 5.8 APPLICATION: Input Validation 6 LOOPS 6.1 The while Loop 6.2 PROBLEM SOLVING: Hand-Tracing 6.3 The for Loop ST 1 Variables Declared in a for Loop Header 6.4 The do Loop 6.5 APPLICATION: Processing Sentinel Values ST 2 Redirection of Input and Output ST 3 The “Loop and a Half” Problem ST 4 The break and continue Statements 6.6 PROBLEM SOLVING: Storyboards 6.7 Common Loop Algorithms Sum and Average Value Counting Matches Finding the First Match Prompting Until a Match is Found Maximum and Minimum Comparing Adjacent Values HT 1 Writing a Loop WE 1 Credit Card Processing 6.8 Nested Loops WE 2 Manipulating the Pixels in an Image 6.9 APPLICATION: Random Numbers and Simulations Generating Random Numbers The Monte Carlo Method 6.10 Using a Debugger HT 2 Debugging WE 3 A Sample Debugging Session 7 ARRAYS AND ARRAY LISTS 7.1 Arrays Declaring and Using Arrays Array References Using Arrays with Methods Partially Filled Arrays ST 1 Methods with a Variable Number of Arguments 7.2 The Enhanced for Loop 7.3 Common Array Algorithms Filling Sum and Average Value Maximum and Minimum Element Separators Linear Search Removing an Element Inserting an Element Swapping Elements Copying Arrays Reading Input ST 2 Sorting with the Java Library 7.4 PROBLEM SOLVING: Adapting Algorithms HT 1 Working with Arrays WE 1 Rolling the Dice 7.5 PROBLEM SOLVING: Discovering Algorithms by Manipulating Physical Objects 7.6 Two-Dimensional Arrays Declaring Two-Dimensional Arrays Accessing Elements Locating Neighboring Elements Accessing Rows and Columns Two-Dimensional Array Parameters WE 2 A World Population Table ST 3 Two-Dimensional Arrays with Variable Row Lengths ST 4 Multidimensional Arrays 7.7 Array Lists Declaring and Using Array Lists Using the Enhanced for Loop with Array Lists Copying Array Lists Wrappers and Auto-boxing Using Array Algorithms with Array Lists Storing Input Values in an Array List Removing Matches Choosing Between Array Lists and Arrays ST 5 The Diamond Syntax 7.8 Regression Testing 8 DESIGNING CLASSES 8.1 Discovering Classes 8.2 Designing Good Methods Providing a Cohesive Public Interface Minimizing Dependencies Separating Accessors and Mutators Minimizing Side Effects ST 1 Call by Value and Call by Reference 8.3 PROBLEM SOLVING: Patterns for Object Data Keeping a Total Counting Events Collecting Values Managing Properties of an Object Modeling Objects with Distinct States Describing the Position of an Object 8.4 Static Variables and Methods ST 2 Alternative Forms of Instance and Static Variable Initialization ST 3 Static Imports 8.5 PROBLEM SOLVING: Solve a Simpler Problem First 8.6 Packages Organizing Related Classes into Packages Importing Packages Package Names Packages and Source Files ST 4 Package Access HT 1 Programming with Packages 8.7 Unit Test Frameworks 9 INHERITANCE 9.1 Inheritance Hierarchies 9.2 Implementing Subclasses 9.3 Overriding Methods ST 1 Calling the Superclass Constructor 9.4 Polymorphism ST 2 Dynamic Method Lookup and the Implicit Parameter ST 3 Abstract Classes ST 4 Final Methods and Classes ST 5 Protected Access HT 1 Developing an Inheritance Hierarchy WE 1 Implementing an Employee Hierarchy for Payroll Processing 9.5 OBJECT: The Cosmic Superclass Overriding the toString Method The equals Method The instanceof Operator ST 6 Inheritance and the toString Method ST 7 Inheritance and the equals Method 10 INTERFACES 10.1 Using Interfaces for Algorithm Reuse Discovering an Interface Type Declaring an Interface Type Implementing an Interface Type Comparing Interfaces and Inheritance ST 1 Constants in Interfaces ST 2 Nonabstract Interface Methods 10.2 Working with Interface Types Converting from Classes to Interfaces Invoking Methods on Interface Variables Casting from Interfaces to Classes WE 1 Investigating Number Sequences 10.3 The Comparable Interface ST 3 The clone Method and the Cloneable Interface 10.4 Using Interfaces for Callbacks ST 4 Lambda Expressions ST 5 Generic Interface Types 10.5 Inner Classes 10.6 Mock Objects 10.7 Event Handling Listening to Events Using Inner Classes for Listeners 10.8 Building Applications with Buttons 10.9 Processing Timer Events 10.10 Mouse Events ST 6 Keyboard Events ST 7 Event Adapters 11 INPUT/OUTPUT AND EXCEPTION HANDLING 11.1 Reading and Writing Text Files ST 1 Reading Web Pages ST 2 File Dialog Boxes ST 3 Character Encodings 11.2 Text Input and Output Reading Words Reading Characters Classifying Characters Reading Lines Scanning a String Converting Strings to Numbers Avoiding Errors When Reading Numbers Mixing Number, Word, and Line Input Formatting Output ST 4 Regular Expressions ST 5 Reading an Entire File 11.3 Command Line Arguments HT 1 Processing Text Files WE 1 Analyzing Baby Names 11.4 Exception Handling Throwing Exceptions Catching Exceptions Checked Exceptions Closing Resources Designing Your Own Exception Types ST 6 Assertions ST 7 The try/finally Statement 11.5 APPLICATION: Handling Input Errors 12 OBJECT-ORIENTED DESIGN 12.1 Classes and Their Responsibilities Discovering Classes The CRC Card Method 12.2 Relationships Between Classes Dependency Aggregation Inheritance HT 1 Using CRC Cards and UML Diagrams in Program Design ST 1 Attributes and Methods in UML Diagrams ST 2 Multiplicities ST 3 Aggregation, Association, and Composition 12.3 APPLICATION: Printing an Invoice Requirements CRC Cards UML Diagrams Method Documentation Implementation WE 1 Simulating an Automatic Teller Machine 13 RECURSION 13.1 Triangle Numbers HT 1 Thinking Recursively WE 1 Finding Files 13.2 Recursive Helper Methods 13.3 The Efficiency of Recursion 13.4 Permutations 13.5 Mutual Recursion 13.6 Backtracking WE 2 Towers of Hanoi 14 SORTING AND SEARCHING 14.1 Selection Sort 14.2 Profiling the Selection Sort Algorithm 14.3 Analyzing the Performance of the Selection Sort Algorithm ST 1 Oh, Omega, and Theta ST 2 Insertion Sort 14.4 Merge Sort 14.5 Analyzing the Merge Sort Algorithm ST 3 The Quicksort Algorithm 14.6 Searching Linear Search Binary Search 14.7 PROBLEM SOLVING: Estimating the Running Time of an Algorithm Linear Time Quadratic Time The Triangle Pattern Logarithmic Time 14.8 Sorting and Searching in the Java Library Sorting Binary Search Comparing Objects ST 4 The Comparator Interface ST 5 Comparators with Lambda Expressions WE 1 Enhancing the Insertion Sort Algorithm 15 THE JAVA COLLECTIONS FRAMEWORK 15.1 An Overview of the Collections Framework 15.2 Linked Lists The Structure of Linked Lists The LinkedList Class of the Java Collections Framework List Iterators 15.3 Sets Choosing a Set Implementation Working with Sets 15.4 Maps ST 1 Updating Map Entries HT 1 Choosing a Collection WE 1 Word Frequency ST 2 Hash Functions 15.5 Stacks, Queues, and Priority Queues Stacks Queues Priority Queues 15.6 Stack and Queue Applications Balancing Parentheses Evaluating Reverse Polish Expressions Evaluating Algebraic Expressions Backtracking ST 3 Reverse Polish Notation WE 2 Simulating a Queue of Waiting Customers 16 BASIC DATA STRUCTURES 16.1 Implementing Linked Lists The Node Class Adding and Removing the First Element The Iterator Class Advancing an Iterator Removing an Element Adding an Element Setting an Element to a Different Value Efficiency of Linked List Operations ST 1 Static Classes WE 1 Implementing a Doubly-Linked List 16.2 Implementing Array Lists Getting and Setting Elements Removing or Adding Elements Growing the Internal Array 16.3 Implementing Stacks and Queues Stacks as Linked Lists Stacks as Arrays Queues as Linked Lists Queues as Circular Arrays 16.4 Implementing a Hash Table Hash Codes Hash Tables Finding an Element Adding and Removing Elements Iterating over a Hash Table ST 2 Open Addressing 17 TREE STRUCTURES 17.1 Basic Tree Concepts 17.2 Binary Trees Binary Tree Examples Balanced Trees A Binary Tree Implementation WE 1 Building a Huffman Tree 17.3 Binary Search Trees The Binary Search Property Insertion Removal Efficiency of the Operations 17.4 Tree Traversal Inorder Traversal Preorder and Postorder Traversals The Visitor Pattern Depth-First and Breadth-First Search Tree Iterators 17.5 Red-Black Trees Basic Properties of Red-Black Trees Insertion Removal WE 2 Implementing a Red-Black Tree 17.6 Heaps 17.7 The Heapsort Algorithm 18 GENERIC CLASSES 18.1 Generic Classes and Type Parameters 18.2 Implementing Generic Types 18.3 Generic Methods 18.4 Constraining Type Parameters ST 1 Wildcard Types 18.5 Type Erasure ST 2 Reflection WE 1 Making a Generic Binary Search Tree Class 19 STREAM PROCESSING 19.1 The Stream Concept 19.2 Producing Streams 19.3 Collecting Results ST 1 Infinite Streams 19.4 Transforming Streams 19.5 Lambda Expressions ST 2 Method and Constructor References ST 3 Higher-Order Functions ST 4 Higher-Order Functions and Comparators 19.6 The Optional Type 19.7 Other Terminal Operations 19.8 Primitive-Type Streams Creating Primitive-Type Streams Mapping a Primitive-Type Stream Processing Primitive-Type Streams 19.9 Grouping Results 19.10 Common Algorithms Revisited Filling Sum, Average, Maximum, and Minimum Counting Matches Element Separators Linear Search Comparing Adjacent Values HT 1 Working with Streams WE 1 Word Properties WE 2 A Movie Database 20 GRAPHICAL USER INTERFACES 20.1 Layout Management Using Layout Managers Achieving Complex Layouts Using Inheritance to Customize Frames ST 1 Adding the main Method to the Frame Class 20.2 Processing Text Input Text Fields Text Areas 20.3 Choices Radio Buttons Check Boxes Combo Boxes HT 1 Laying Out a User Interface WE 1 Programming a Working Calculator 20.4 Menus 20.5 Exploring the Swing Documentation 21 ADVANCED INPUT/ OUTPUT* (ETEXT ONLY) 21.1 Readers, Writers, and Input/Output Streams 21.2 Binary Input and Output 21.3 Random Access 21.4 Object Input and Output Streams HT 1 Choosing a File Format 21.5 File and Directory Operations Paths Creating and Deleting Files and Directories Useful File Operations Visiting Directories 22 MULTITHREADING* (ETEXT ONLY) 22.1 Running Threads ST 1 Thread Pools 22.2 Terminating Threads 22.3 Race Conditions 22.4 Synchronizing Object Access 22.5 Avoiding Deadlocks ST 2 Object Locks and Synchronized Methods ST 3 The Java Memory Model 22.6 APPLICATION Algorithm Animation 23 INTERNET NETWORKING* (ETEXT ONLY) 23.1 The Internet Protocol 23.2 Application Level Protocols 23.3 A Client Program 23.4 A Server Program HT 1 Designing Client/Server Programs 23.5 URL Connections 24 RELATIONAL DATABASES* (ETEXT ONLY) 24.1 Organizing Database Information Database Tables Linking Tables Implementing Multi-Valued Relationships ST 1 Primary Keys and Indexes 24.2 Queries Simple Queries Selecting Columns Selecting Subsets Calculations Joins Updating and Deleting Data 24.3 Installing a Database 24.4 Database Programming in Java Connecting to the Database Executing SQL Statements Analyzing Query Results Result Set Metadata 24.5 APPLICATION Entering an Invoice ST 2 Transactions ST 3 Object-Relational Mapping WE 1 Programming a Bank Database 25 XML* (ETEXT ONLY) 25.1 XML Tags and Documents Advantages of XML Differences Between XML and HTML The Structure of an XML Document HT 1 Designing an XML Document Format 25.2 Parsing XML Documents 25.3 Creating XML Documents HT 2 Writing an XML Document ST 1 Grammars, Parsers, and Compilers 25.4 Validating XML Documents Document Type Definitions Specifying a DTD in an XML Document Parsing and Validation HT 3 Writing a DTD ST 2 Schema Languages ST 3 Other XML Technologies Appendix A THE BASIC LATIN AND LATIN-1 SUBSETS OF UNICODE Appendix B JAVA OPERATOR SUMMARY A Appendix C JAVA RESERVED WORD SUMMARY A Appendix D THE JAVA LIBRARY Appendix E JAVA LANGUAGE CODING GUIDELINES Appendix F TOOL SUMMARY (ETEXT ONLY) Appendix G NUMBER SYSTEMS (ETEXT ONLY) Appendix H UML SUMMARY (ETEXT ONLY) Appendix I JAVA SYNTAX SUMMARY (ETEXT ONLY) Appendix J HTML SUMMARY (ETEXT ONLY) GLOSSARY INDEX CREDITS QUICK REFERENCE
|