Programming for Problem Solving, as per AICTE
ISBN: 9788126519927
460 pages
eBook also available for institutional users
For more information write to us at: acadmktg@wiley.com
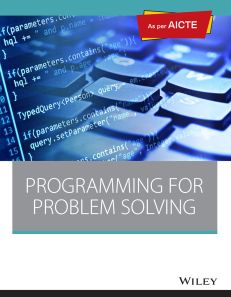
Description
This book is designed to meet the requirements of students having very little knowledge of computers, and journeys them from the basic fundaments of computers through application of problem-solving techniques using C programming concepts. The book also includes laboratory manual for practical applications of the theoretical concepts on problem solving through C programming. Useful appendices in the book serve as a good reference tools for related chapters.
Preface
Chapter 1 Introduction to Computers
1.1 Introduction
1.2 Evolution of Computers and Computer Generations
1.3 Classification of Computers
1.4 Basic Computer Organization
1.5 Types of Number Systems
1.6 Signed Binary Numbers
1.7 Binary Arithmetic Operations
1.8 Octal and Hexadecimal Arithmetic Operations
1.9 Coding Standards to Represent Stored Data
1.10 Operating System
1.11 Computer Applications
Chapter 2 Introduction to Programming
2.1 Introduction
2.2 Problem Formulation and Planning a Program
2.3 Steps for Program Development
2.4 Problem Solving Techniques
2.5 Program Control Structures
2.6 Evolution of Programming Languages
2.7 Program Methodology
2.8 Programming Models
Chapter 3 Basics of Programming in C 71
3.1 Introduction
3.2 C Character Set and Tokens
3.3 Operators and Expressions
3.4 Structure of a C program
3.5 Managing Input and Output Operations
3.6 Decision Making Statements
3.7 Looping and Branching Statements
3.8 Solving Simple Scientific and Statistical Problems
Chapter 4 Arrays and Strings
4.1 Introduction
4.2 One-Dimensional Array
4.3 Multidimensional Arrays
4.4 Strings and String Functions
Chapter 5 Functions and Pointers
5.1 Introduction
5.2 User Defined Functions
5.3 Types of Functions based on their Return Type and Function Call
5.4 Arrays and Functions
5.5 Pointers
5.6 Dynamic Memory Allocation
Chapter 6 Structures and Unions
6.1 Introduction
6.2 Structure Declaration and Accessing Structure Elements
6.3 Initialization of a Structure
6.4 Array and Structures
6.5 Nested Structures
6.6 Structure and Function
6.7 Pointer to Structures
6.8 Unions
6.9 Storage Classes
6.10 Preprocessor Directives and Standard Library Functions
Chapter 7 Data Structures and Algorithms
7.1 Introduction
7.2 Stack
7.3 Queue
7.4 Linked List
7.5 Trees
7.6 Algorithms
7.7 Hashing
7.8 Binary Heap
7.9 Searching and Sorting
7.10 Finding Roots of Quadratic Equation
Chapter 8 File Handling in C
8.1 Introduction
8.2 Basic Terminology Associated with Files
8.3 Types of Files
8.4 Streams and Files
8.5 File System Structures
8.6 Various Types of File Access Methods
8.7 Input and Output Operations on Files and Standard Devices
8.8 File Operations
8.9 Error Handling in Files
8.10 Command Line Arguments
Points to Remember
Multiple Choice Questions
Review Questions
Programming Questions
Answer Key
Appendix A ASCII Set
Appendix B Header Files and Standard Library Functions
Appendix C Syntax Statements
Appendix D Predicting Outputs and Identifying Errors
Appendix E Programming for Problem Solving Laboratory