Object Oriented Programming using Java: As per CE, CSE, IT and ICT - B.E 5th semester syllabus of GTU
ISBN: 9789351198178
400 pages
eBook also available for institutional users
For more information write to us at: acadmktg@wiley.com
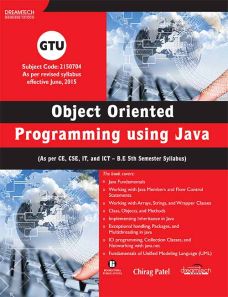
Description
This book introduces the Java programming language and explains how to create Java applications and work with UML. It also discusses various Java programming concepts, such as Object Oriented Programming (OOP), arrays as data structure, inheritance, multithreaded programming and networking. Moreover, this book also covers the fundamentals of UML.
Introduction
Chapter 1: Introduction to Java
1.1 Introducing Object-Oriented Programming
1.2 Evolution of Java
1.3 Comparing Java with C++
1.4 Features of Java
1.5 Exploring New Features of Java SE 8.0
1.6 Introducing the Java Environment
1.7 Developing a Simple Java Program
Chapter 2: Fundamental Concepts in Java Programming
2.1 Working with Java Tokens
2.2 Operator Precedence and Associativity
2.3 Declaring Variables
2.4 Introducing Data Types
2.5 Control Statements
Chapter 3: Working with Arrays, Strings, String Buffer Class and Wrapper Classes
3.1 Working with Arrays
3.2 Working with Strings
3.3 Using the String Buffer Class
3.4 Using Command Line Arguments
3.5 Using the Wrapper Classes
Chapter 4: Working with Classes, Objects and Methods
4.1 Working with Classes
4.2 Working with Objects
4.3 Explaining Constructors
4.4 Declaring Methods
4.5 Recursion in Java
4.6 Working with Abstract Class
4.7 Working with Nested Classes
4.8 Working with the Inner Class
4.9 Working with the Anonymous Inner Class
Chapter 5: Inheritance and Interfaces in Java
5.1 Understanding Inheritance
5.2 Constructors in Inheritance
5.3 Multilevel Inheritance
5.4 Using the final Keyword
5.5 Working with Interfaces in Java
5.6 Understanding Dynamic Method Dispatch
5.7 Understanding the Java Object Class
Chapter 6: Working with Packages and Exceptions
6.1 Understanding Packages in Java
6.2 JAR Files
6.3 Defining Java API Packages
6.4 Handling Exceptions
Chapter 7: Working with Thread
7.1 An Overview of Threads
7.2 Defining a Thread
7.3 Instantiating a Thread
7.4 Starting a Thread
7.5 Thread States and Transitions
7.6 Code Synchronization
7.7 Thread Interaction
Chapter 8: Working with Streams
8.1 Introduction to Stream
8.2 Introduction to NIO
8.3 Working with Stream Classes
8.4 Working with Files
8.5 Working with Buffers
8.6 Working with Character Arrays
8.7 Working with the Print Writer Class
8.8 Working with the Stream Tokenizer Class
8.9 Implementing the Serializable Interface
8.10 Working with the Console Class
8.11 Printing with the Formatter Class
8.12 Scanning Input with the Scanner class
Chapter 9: Collection Classes
9.1 Collection Interfaces
9.2 Classes of Collection
9.3 Legacy Classes
9.4 The Enumeration
Chapter 10: Networking with Java.net
10.1 Introduction to Networking
10.2 Networking Enhancements in Java SE 8
10.3 Client-Server Networking
10.4 Proxy Servers
10.5 Domain Name Service
10.6 Understanding Networking Interfaces and Classes in the java.net Package
10.7 Internet Addressing
10.8 Understanding Sockets in Java
10.9 Understanding the URL Class
10.10 Understanding the URI Class
10.11 Working with Datagrams
Chapter 11: Introduction to Object Orientation and Modeling Concepts
11.1 Object Orientation
11.2 Object-Oriented Development
11.3 Object-Oriented Themes
11.4 Concepts of Modeling and Modeling as a Design Technique
11.5 The Three Models
11.6 Relationship among the Models
Chapter 12: Class Modeling and Advanced Class Modeling
12.1 Concepts of Object and Class
12.2 Associations and Links
12.3 Aggregation
12.4 Generalization and Inheritance
12.5 Abstract Class
12.6 Multiple Inheritances
12.7 Metadata
12.8 Constraints
12.9 Derived Data and Packages
Chapter 13: State Modelling
13.1 Events
13.2 States
13.3 Transitions and Conditions
13.4 State Diagram
13.5 State Diagram Behavior
Chapter 14: Interaction Modeling
14.1 Use Case Models
14.2 Sequence Model
14.3 Activity Model
14.4 Collaboration Diagram
14.5 Difference and Similarities between Sequence and Collaboration Diagram