Full Stack Java Development with Spring MVC, Hibernate, jQuery, and Bootstrap
ISBN: 9788126519910
712 pages
For more information write to us at: acadmktg@wiley.com
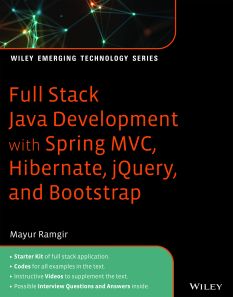
Description
Full Stack Java Development is a great way to start one's journey to becoming a full stack developer. Any student will find this book very helpful to learn about the essential technologies and ecosystem of web application development. Web technologies like HTML, JQuery, Bootstrap, Webservices, etc. are well explained in the book. The exploration is not just limited to theoretical knowledge as the book also has add-ons to improve one's understanding of the subject. This book will greatly help students prepare to become full stack developers.
Chapter 1 Introduction to Full Stack Development
1.1 Introduction
1.2 What is Full Stack Web Development?
1.3 Introduction to Web Application Development
1.4 Front-End Technologies
1.5 Back-End Technologies (Server-Side)
1.6 Introduction to Back-end Development with Java 11
1.7 Introduction to Model View Controller (MVC)
1.8 Introduction to Web Services: API-Based Architecture with REST
1.9 Communication Between Front-End and Back-End
1.10 Introduction to Object Relational Mapping (ORM) with Hibernate
Chapter 2 Getting Started with Full Stack Development: A Project Idea
2.1 Introduction
2.2 Project Outline
2.3 What is E-Commerce?
2.4 Required Entities
2.5 Entity Relationship Diagram
2.6 UML Class Diagram
2.7 Flowchart
2.8 Front-End Page Flow Design
2.9 Back-End Web Services API Endpoints
Chapter 3 Introduction to Hyper Text Markup Language
3.1 Overview of HTML
3.2 Getting Started with HTML Code
3.3 Important Components of HTML
3.4 Text Formatting Tags
3.5 Quotations
3.6 Comments
3.7 Links
3.8 Images
3.9 Tables
3.10 Lists
3.11 Attributes to Style HTML Elements
Chapter 4 Introduction to Cascading Style Sheets
4.1 Introduction
4.2 Overview of CSS
4.3 Relationship Between HTML and CSS
4.4 How Does CSS Work?
4.5 Syntax
4.6 Different Methods to Integrate CSS with HTML
4.7 Colors
4.8 Backgrounds in CSS
4.9 Setting up Height and Width of an Element
4.10 Box Model
4.11 CSS Outline
4.12 Text in CSS
4.13 Fonts
4.14 Links in CSS
4.15 Lists in CSS
4.16 Tables in CSS
4.17 Responsiveness
4.18 Position Property in CSS
4.19 Navigation Bars
4.20 Dropdown
4.21 Forms
Chapter 5 Introduction to jQuery
5.1 Overview of jQuery
5.2 Configuration of jQuery
5.3 Syntax
5.4 Selectors
5.5 Events
5.6 Effects
5.7 Working with HTML
5.8 jQuery with CSS
5.9 Traversing
Chapter 6 Introduction to Bootstrap
6.1 Overview of Bootstrap
6.2 Structure of a Bootstrap-enabled Webpage
6.3 Grids
6.4 Typography
6.5 Colors
6.6 Images
6.7 Jumbotron
6.8 Alerts
6.9 Buttons
6.10 Button Groups
6.11 Progress Bars
6.12 Pagination
6.13 Cards
6.14 Navigation Menus
6.15 Navigation Bar
6.16 Forms
6.17 Carousel
6.18 Media Objects
Chapter 7 Build Pages for MyEShop with HTML and CSS
7.1 Setting up Environment
7.2 Identify the Pages
7.3 Getting Started with HTML Pages
7.4 Adding CSS to the HTML Page
Chapter 8 Use of jQuery on HTML CSS
8.1 Getting Started with jQuery
8.2 Home Page with jQuery
Chapter 9 Use of Bootstrap to Make HTML Responsive
9.1 Setting up Environment
Chapter 10 Introduction to Java Language
10.1 Overview of Java
10.2 Basic Java Concepts
10.3 Principles of Object-Oriented Programming in Java
10.4 Programming in Java
10.5 Java Packages
10.6 New Features in Java 9
10.7 Eclipse IDE for Programming
Chapter 11 Language Syntax and Elements of Language
11.1 Building Blocks of Java
11.2 Calling the Main Method
11.3 String Options
11.4 Arrays
11.5 Enums
11.6 Wrapper Classes
11.7 Autoboxing and Unboxing
11.8 Developing Logic
11.9 Control Flow
11.10 Loops
11.11 Branching
Chapter 12 Object-Oriented Programming
12.1 Introduction
12.2 Object-Oriented Programming Principles
12.3 Object-Oriented Programming Principles in Application
12.4 Understanding an Interface
12.5 Overriding and Overloading
12.6 Coupling and Cohesion
12.7 Implementation in Java
12.8 Future of Object-Oriented Programming
12.9 Understanding the World
Chapter 13 Generics and Collections
13.1 Introduction
13.2 Generic Programming
13.3 Collections
13.4 Implementing Collection Classes
13.5 List of Key Methods for Arrays and Collections
Chapter 14 Error Handling
14.1 Introduction
14.2 Understanding Error Handling
14.3 Logical Errors
14.4 Syntactical Errors
14.5 Semantic Errors
14.6 Importance of Error Handling
14.7 Checked verses Runtime Exceptions
Chapter 15 Garbage Collection
15.1 Introduction
15.2 Garbage Collection in Java
15.3 Major Garbage Collection
15.4 G1 and CMS Garbage Collectors
15.5 Advantages of Garbage Collection in Java
15.6 Making Objects Eligible for Garbage Collection
15.7 JEP 318 – Epsilon: A No-Op Garbage Collector
Chapter 16 Strings, I/O Operations, and File Management
16.1 Introduction
16.2 Role of Strings in Java
16.3 Types of String Operations
16.4 StringBuilder and String Buffer Explained
16.5 Java I/O
16.6 File Management in Java
Chapter 17 Data Structure and Integration in Program
17.1 Introduction
17.2 Introduction to Data Structures
17.3 Classification of Data Structures
Chapter 18 Lambdas and Functional Programming
18.1 Introduction
18.2 Functional Programming
18.3 Functional Programming in Java
18.4 Object-Oriented versus Functional Programming
18.5 Lambdas
18.6 Date and Time API
Chapter 19 Multithreading and Reactive Programming
19.1 Introduction
19.2 Reactive Programming
19.3 Reactive Programming
19.4 What is Multithreading?
19.5 Concurrency
19.6 Understanding Deadlock
19.7 Concurrent Data Structures
19.8 Multithreading Examples
19.9 Designing Concurrent Java Programs
Chapter 20 Introduction to Spring and Spring MVC
20.1 Spring Framework
20.2 Spring Architecture
20.3 Spring MVC
20.4 Interception
20.5 Chain of Resolvers
20.6 View Resolution
20.7 Multiple View Pages
20.8 Multiple Controllers
20.9 Model Interface
20.10 RequestParam
20.11 Form Tag Library
20.12 Form Text Field
20.13 CRUD Example
20.14 File Upload in Spring MVC
20.15 Validation in Spring MVC
20.16 Validation with Regular Expression
20.17 Validation with Numbers
Chapter 21 Introduction to Hibernate
21.1 Introduction
21.2 Architecture
21.3 Installation and Configuration
21.4 Java Objects in Hibernate
21.5 Inheritance Mapping
21.6 Collection Mapping
21.7 Mapping with Map
21.8 Hibernate Query Language
21.9 Caching
21.10 Spring Integration
Chapter 22 Develop Web Services for the APIs
22.1 Setting up Environment
22.2 Creating a New Project
22.3 Creating Models
22.4 Creating Data Access Object
22.5 Creating Controller
Chapter 23 Develop Models with Hibernate
23.1 Installing MySQL
23.2 Create Database and Tables
23.3 Making DAO to Perform CRUD
Summary
Multiple-Choice Questions
Review Questions
Exercises
Project Idea
Recommended Readings
Annexure A: Consuming Web Services
Annexure B: Possible Interview Questions and Answers
Annexure C: Answers to Objective Type Questions
Index